Next step is to connect the Angular application with Firebase. This should be rather straight forward.
- add the npm package
- configure the environment
- test the connectivity
Add Firebase modules
ng add @angular/fire
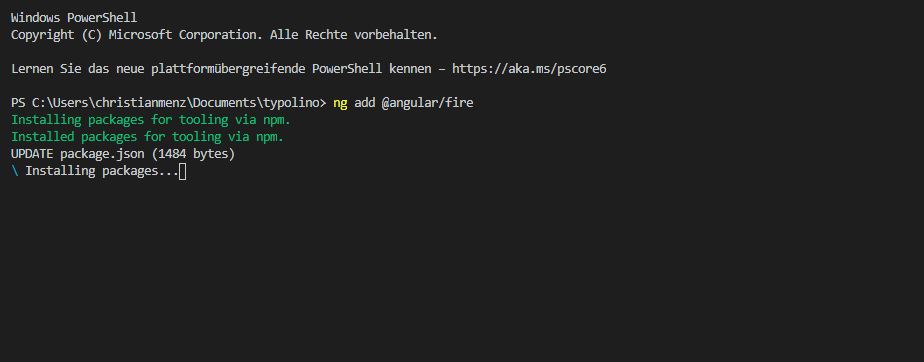
Actually I just realized that adding @angular/fire will do some stuff we already did before. Not an issue but I had to cleanup firebase.json as it added another hosting configuration automatically.

In addition it registered a new ng command to deploy the application. This is maybe very helpful but to be honest I kind of dislike this magic. At least it would be nice if the commands tell me what they changed. Well I got a list of files that where updated, but it could be a bit more verbose.

Configuration
Now that I have the library added I need to add a web app (Firebase console). The steps are easy and the ultimate goal is to get the configuration parameters which I finally added to the environment.ts file.
The process is very well described here.
Setup Angular
Last but not least I have to add the Angular modules to the application. I’m using VSCode. In my opinion the best editor out there right now. It offers great support for TypeScript and Angular.
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AngularFireModule } from '@angular/fire'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import { environment } from 'src/environments/environment'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, AppRoutingModule, AngularFireModule.initializeApp(environment.firebase) ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
I personally don’t like the way I have to import files by relative path in TypeScript code. Notice the way I imported the environment file. Therefore I like to set path aliases. Here is my tsconfig.json after the change:
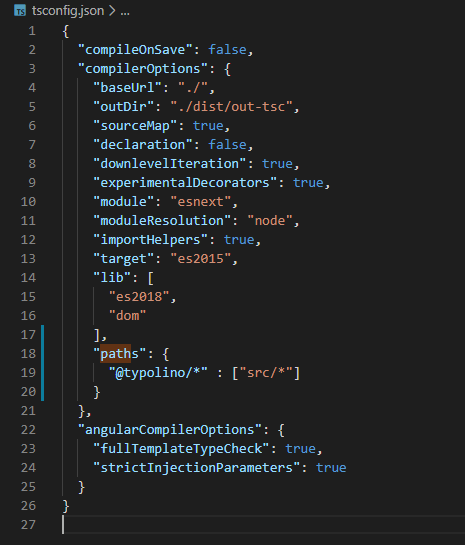
And now I can change the import from above to (anyhwere I need it!):
import { environment } from '@typolino/environments/environment';
Next step is to connect to a collection and try to read some data.
Reading some data
For this I just use the initially created app.component.
<div> {{data | async | json}} </div>
import { Component, OnInit } from '@angular/core'; import { AngularFirestore } from '@angular/fire/firestore'; import { Observable } from 'rxjs'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.less'] }) export class AppComponent implements OnInit{ title = 'typolino'; data: Observable<any>; constructor(private firestore: AngularFirestore) { } ngOnInit(): void { this.data = this.firestore.collection('test').valueChanges(); } }
Adding some data
In the Firebase console I created a new collection and aded some random data – just to test everything works fine.
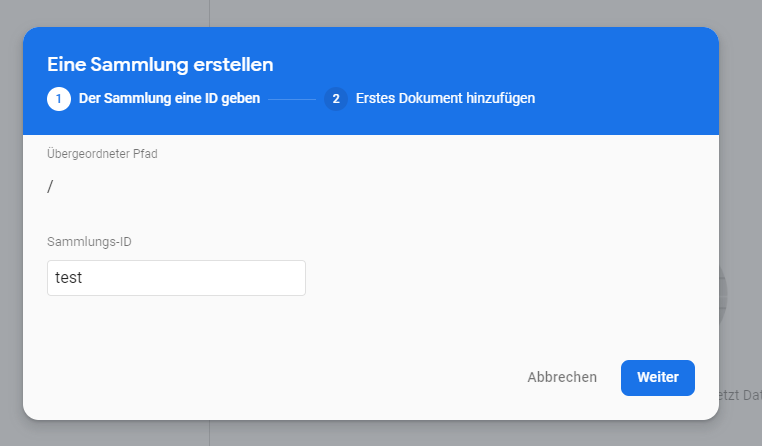

Run the app
Just type ng serve should be sufficient. But I ran in an issue here. I got an error message..

These are the most annoying moments in a software developer’s life. You think you are done and then … nothing works. 🙂
I had to manually add the firebase dependency by running npm install –save firebase. I don’t know why this happened, but I hope it gets fixed.
Now again.. ng serve ..and still no success..
..I need to enable read access on my database. For this we can either change the rules on the Firebase console or change and deploy them directly via the command line.
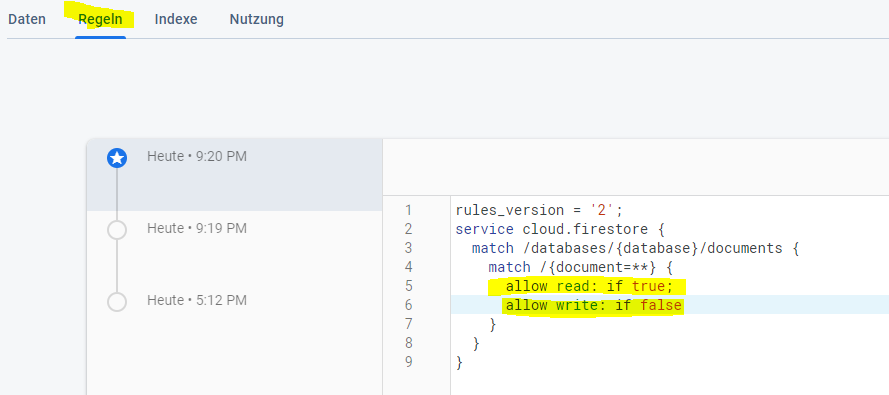
Now again ng serve – and voila!
