I never used animations with Angular. Funny as I remember having used the AngularJS variant quite excessively.
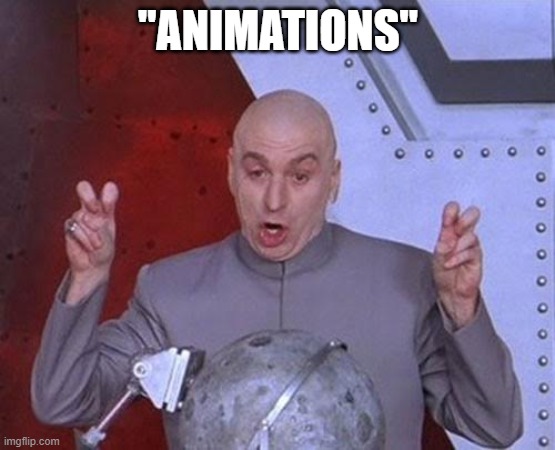
So why not try it out. I’m pretty sure there is a cleverer way of doing it but maybe I just need to get used to the system first.
Seems as if one can simply define the animations as part of a component. Basically you define a trigger and possible transitions. As I wanted to make the image border light up nicely I decided to use keyframe style.
Component({ selector: 'app-lesson', templateUrl: './lesson.component.html', styleUrls: ['./lesson.component.less'], animations: [ trigger('lastInput', [ transition('* => correct', [ animate( '0.4s', keyframes([ style({ backgroundColor: '#21e6c1' }), style({ backgroundColor: '#dbdbdb' }), ]) ), ]), transition('* => wrong', [ animate( '0.4s', keyframes([ style({ backgroundColor: '#ffbd69' }), style({ backgroundColor: '#dbdbdb' }), ]) ), ]), ]), ], })
And this is the template part:
<img [@lastInput]="lastInput" (@lastInput.done)="resetAnimation()" class="lesson__img" [hidden]="!imageLoaded" [src]="currentWord.imageUrl" (load)="loaded()" />
Basically this let’s the image “listen” to changes on the state. Angular evaluates the lastInput and triggers the defined animation. So whenever we set lastInput to either correct or wrong, the animation is triggered. We can trigger now programmatically when a letter is typed:
const newWord = this.userWord + event.key; if (this.currentWord.word.toUpperCase().startsWith(newWord.toUpperCase())) { this.lastInput = 'correct'; this.userWord = newWord; this.checkWord(); } else { this.lastInput = 'wrong'; this.millisSpent += 2_000; // penalty this.highscoreMissed = this.millisSpent > this.lesson.bestTime; } }
To ensure we can play the animation over and over somehow we need to reset the trigger. Really not sure if there isn’t an easier way, but (@lastInput.done)=”resetAnimation()” solves the problem for me.
resetAnimation() { this.lastInput = null; }
Conclusion
I’m really not an expert on the animations part of Angular. But it looks pretty thought through and I feel like I’m in control of the animations.