Finally with Java 14 we get a (preview) feature I had on my wishlist for a long time. It eliminates the need to first test an object via instanceof operator and then (and I asusme it is the 90% case) cast it to exact that class in order to do something with it.
Here is the example directly taken from the JEP site:
if (obj instanceof String s) { // can use s here } else { // can't use s here }
Before we we had to write something like:
if (obj instanceof String) { String s = (String) obj; } else { }
But it gets even better
The binding variable that is introduced by the instanceof operator is not only accessible in the actual true block, but also in the logical extension of the boolean expression. I don’t know how to describe it in a better way.
if (obj instanceof String s && s.contains("hello")) { System.out.println("It contains the magic word!"); }
On the other hand this will not work and if you think it through a bit it also makes sense.
if (obj instanceof String s || s.contains("hello")) { // won't work! System.out.println("It contains the magic word!"); }
Try it out today
You can download early access builds from https://jdk.java.net/14/ (just unzip it somewhere you find it again). No comfort version, without any IDE – it’s fun to use these commands from time to time.
Code
package ch.christianmenz.java14; public class PatternMatching { public static void main(String[] args) { Object obj = "hello world"; if (obj instanceof String s) { System.out.println(s.length()); } } }
Compilation
C:\Users\chris\Documents\Java14\src>c:\Dev\jdk-14\bin\javac -d . -source 14 –enable-preview ch\christianmenz\java14\PatternMatching.java
It is important that you pass the source and the –enable-preview parameters.
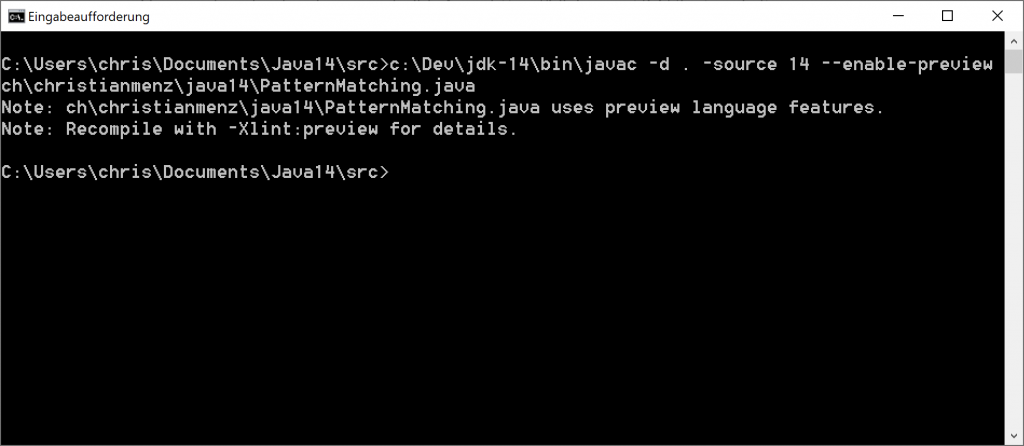
Running
Running is also quite simple (note you don’t have to use pacakges as I did, I just did it for the nostalgic feeling).
C:\Users\chris\Documents\Java14\src>c:\Dev\jdk-14\bin\java –enable-preview -cp . ch.christianmenz.java14.PatternMatching
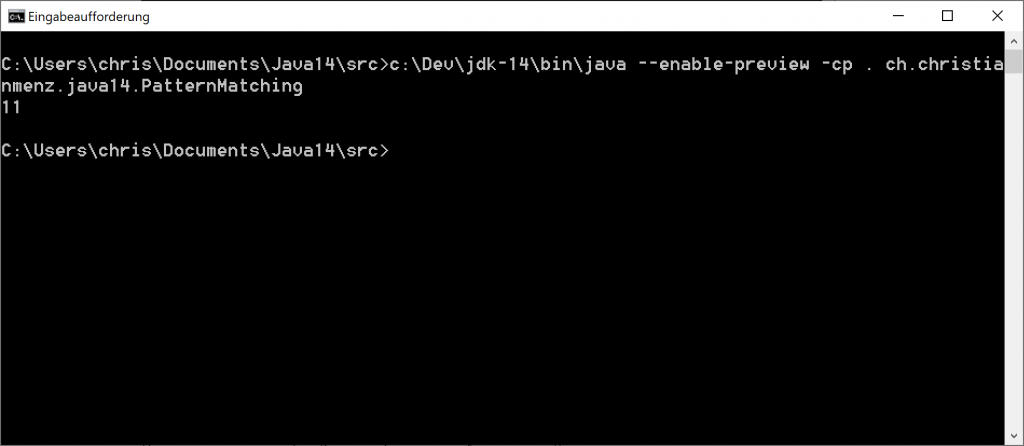
Extra: without manual compilation
If you read about this Java 11 feature, you might wonder if you could also use these preview features: yes you can! Just note you have to pass a double dash this time for the source parameter (I guess because of reasons ;-)).
C:\Users\chris\Documents\Java14\src>c:\Dev\jdk-14\bin\java –enable-preview –source 14 ch\christianmenz\java14\PatternMatching.java
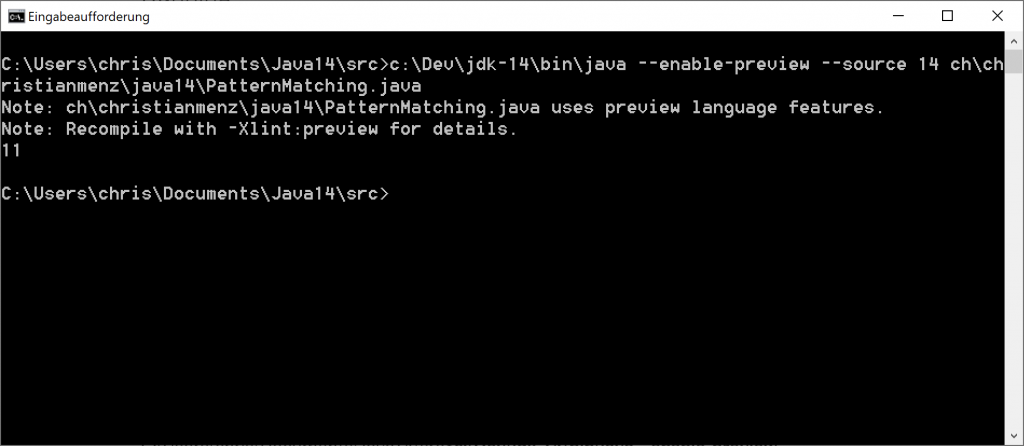
Personal opinion
I’m a bit ambivalent about this feature. I certainly like that some boilerplate code can be eliminated. On the other hand the use of this language construct in combination with more complex expressions might lead to more misunderstandings and harder to understand software. Also keep an eye on this draft JEP, which aims to extend pattern matching to the switch statement: https://openjdk.java.net/jeps/8213076