Since Java 11 we can run Java programs directly from the command line without the need to manually compile them first. Frankly speaking I don’t know if this is a feature I will ever use in my daily work as a developer. But it could be useful to learn Java and provides an easy way to just get started.
Best read http://openjdk.java.net/jeps/330 to learn more about the motivation behind this feautre.
Running .java File
Simply run java HelloWorld.java on your command line and it will compile the class into memory and run it from there.
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello World"); } }

Passing Parameters
You can pass parameters as you normally would when starting your Java program:
java HelloWorld.java Chris
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello " + args[0]); } }
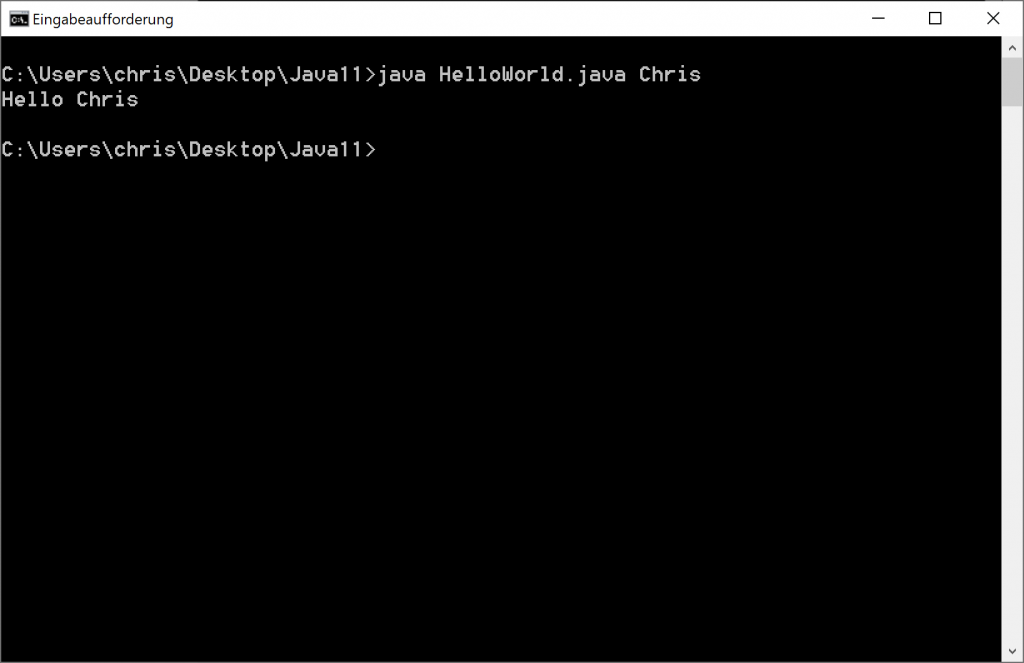
Different Filename
Maybe you don’t want to name your file HelloWorld.java but just hello.j. This is also possible but you need to pass an additional parameter. java –source 11 hello.j
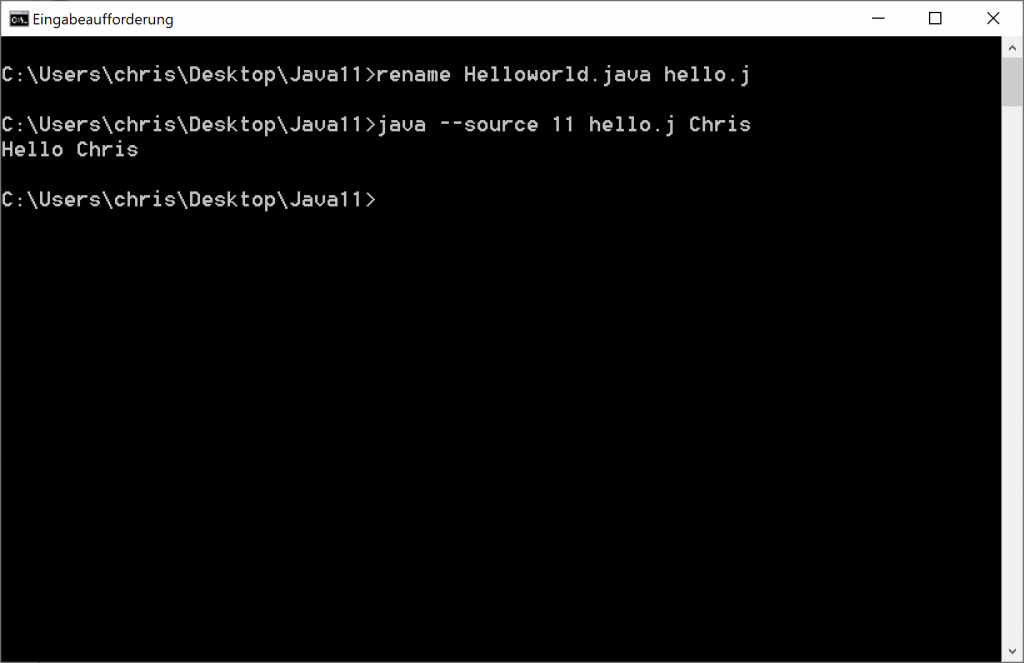
In the example above I just renamed the file and ran it again.
Shebang Files
In addition to just launching Java files direclty you can also add a shebang to the file and execute it directly as a script on Unix like systems (of course you can also use Cygwin or some similar tool on Windows). I just used the Git Bash for the example:
#!java --source 11 public class HelloWorld { public static void main(String[] args) { System.out.println("Hello " + args[0]); } }
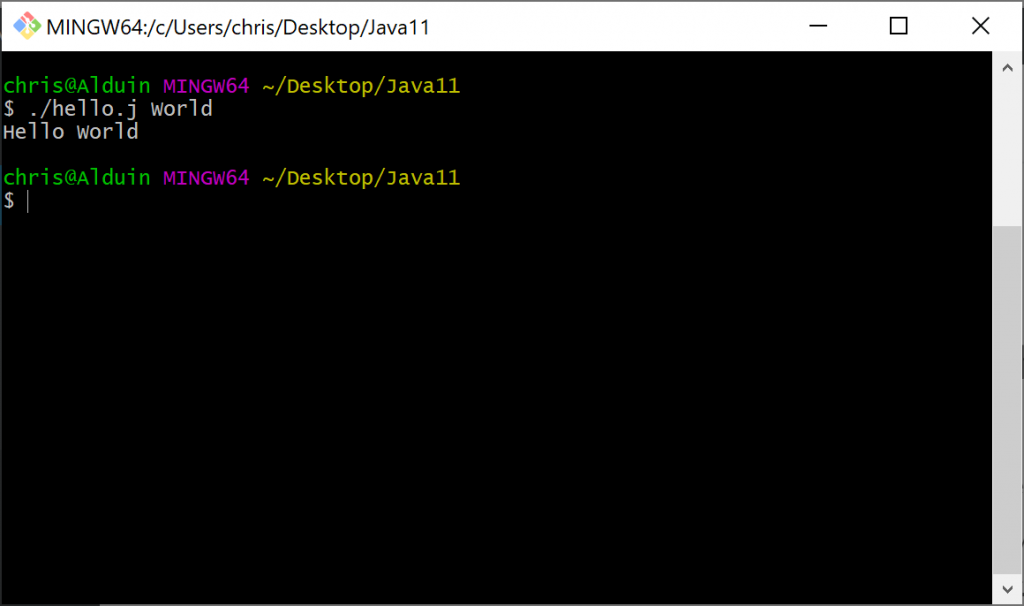