In today’s post, we are going to explore writing unit tests using ChatGPT. We have already built a basic application with React and some scripts to prepare images for the web. The main idea is to input the code into ChatGPT and ask it to generate some tests for us. Let’s see what happens!
Let’s try it
The first time, we simply copied the code into ChatGPT and asked it to generate a unit test. However, when I tried to implement it, it didn’t work at all! Since I was not familiar with the React testing libraries, I felt a bit lost initially. It may not be necessary to share the code here, but I encountered difficulties with mocking the fetch API:
TypeError: Cannot read property 'json' of undefined
19 | const fetchData = async () => {
20 | const response = await fetch("db.json");
> 21 | const json = await response.json();
| ^
22 | setData(json);
23 | };
24 | fetchData();
at fetchData (src/App.js:21:35)
So, I kept asking ChatGPT to help me resolve the issue, but it was a bit frustrating. It explained to me why this error could occur, but the suggested fixes didn’t work. In the end, I had to make significant adjustments to the test myself, as the selection of the correct elements from the screen was not accurate (it provided arbitrary selections). Additionally, I decided to remove the specific open button from the app. On the bright side, I learned about the test library through this process, and I must say, I like it:
- render your component
- read whatever you want from the screen object and assert what you find useful.
Here is the final result:
import { render, screen} from "@testing-library/react";
import App from "./App";
describe("App", () => {
test("renders without errors", () => {
expect(() => render(<App />)).not.toThrow();
});
test("displays image cards with correct data", async () => {
const mockData = {
images: [
{
downloadUrl: "https://example.com/image1.png",
thumbnailUrl: "https://example.com/thumbnail1.png",
title: "Image 1",
alt: "Alt Text 1",
},
{
downloadUrl: "https://example.com/image2.png",
thumbnailUrl: "https://example.com/thumbnail2.png",
title: "Image 2",
alt: "Alt Text 2",
},
],
};
// Mock the fetch request to return the mock data
jest.spyOn(global, "fetch").mockResolvedValue({
json: jest.fn().mockResolvedValue(mockData),
});
render(<App />);
// Verify that the images are rendered with the correct data
await screen.findAllByRole("img");
const images = screen.getAllByRole("img");
expect(images).toHaveLength(mockData.images.length);
mockData.images.forEach((image) => {
const titleElement = screen.getByTitle(image.title);
const altElement = screen.getByAltText(image.alt);
const downloadLink = screen.getByRole("link", {
name: image.alt,
});
expect(titleElement).toBeInTheDocument();
expect(altElement).toBeInTheDocument();
expect(downloadLink).toHaveAttribute("href", image.downloadUrl);
});
});
});
Conclusion
Eventually, it started working. However, I didn’t particularly enjoy the experience because I struggled to identify the correct areas to fix without a solid understanding of the testing library. Nevertheless, I managed to learn how to write simple React tests, which is a positive outcome. Personally, I haven’t found ChatGPT to be very effective in generating tests for React code that actually work.
I would love to hear about your experiences and how you tackled the problems, as well as how productive you felt throughout the process.
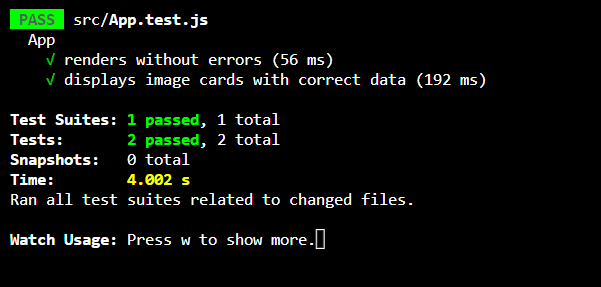